JavaScript Fundamentals
“Sorcery!” exclaimed the Duke of Weasel Town - sorry, the Duke of Weselton as Elsa hurled spears of ice forming a sharp glacial barricade around her. My 17-year-old daughter and I were watching Frozen (probably for the 3 millionth time but who's counting eh?) then it dawned on me… “Elsa is a web developer! (of some sorts)”. Right, I am exaggerating of course but she created one of the most colourful (figuratively speaking) characters in the film - Olaf!
Without realising it, Elsa and Anna first built Olaf when they were kids. They began with a pile of snow and moulded it into spheres, collected a few stones, a couple of sticks and a carrot, all were needed to construct Olaf, pretty much what HTML is for a website.
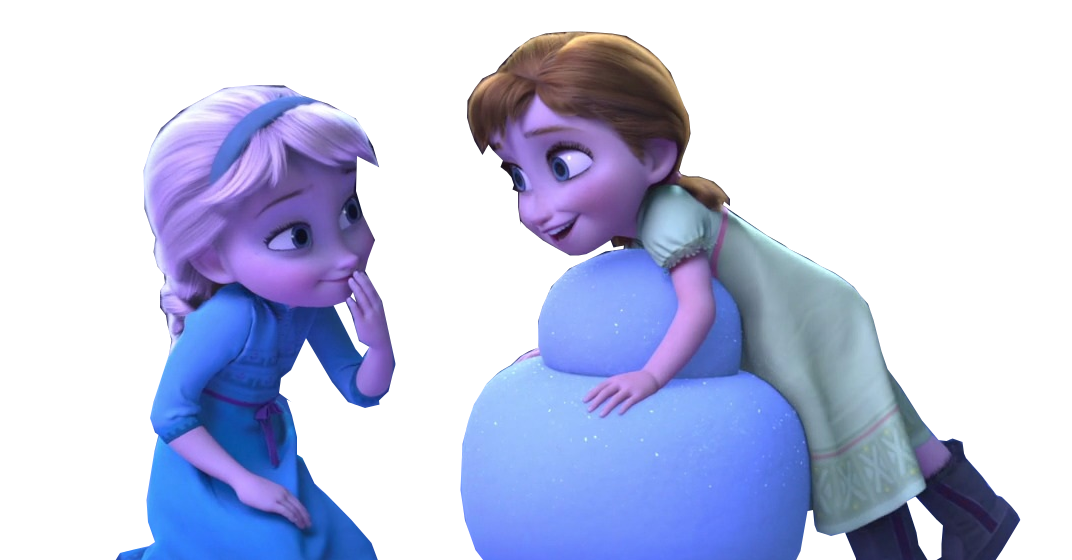
CSS (Cascading Style Sheets) was then applied to position the pieces to their proper place and give colour!
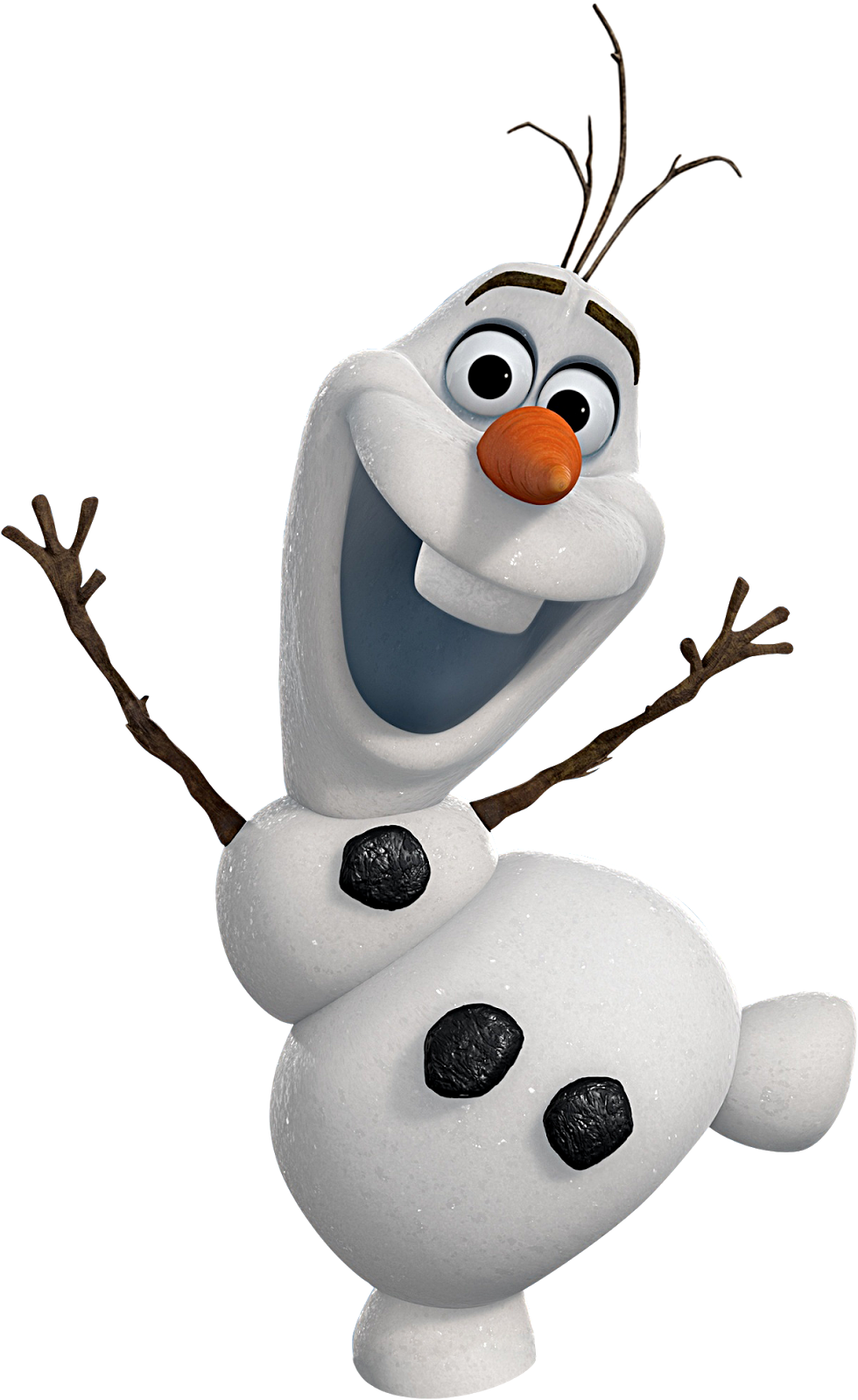
And when Elsa “let it go”, she accidentally breathed life (or JavaScript!) into the dynamic, interactive and summer-loving Olaf.
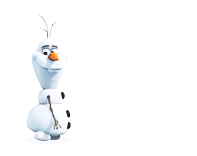
We can perform more magic through the Document Object Model or the DOM. The DOM arranges the elements systematically in the HTML document into a hierarchy of nodes. This gives us the power to reference an object and make changes (add, remove, style, the possibilities are endless! ok, ok, not endless) to it.
There are different ways to pick these objects up, here are some examples. Try clicking on one of the buttons to select Sven or the Frozen sisters. Try hovering your mouse over Kristoff to make him disappear!
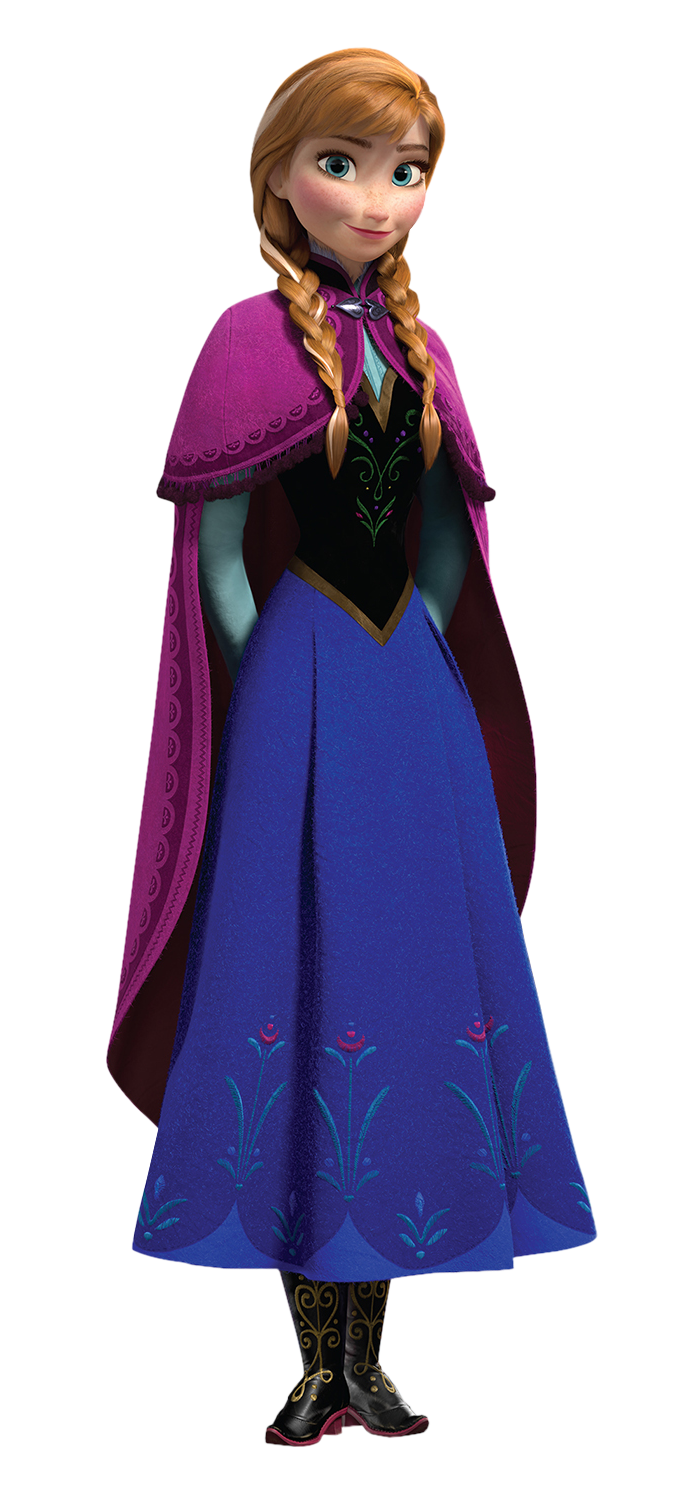
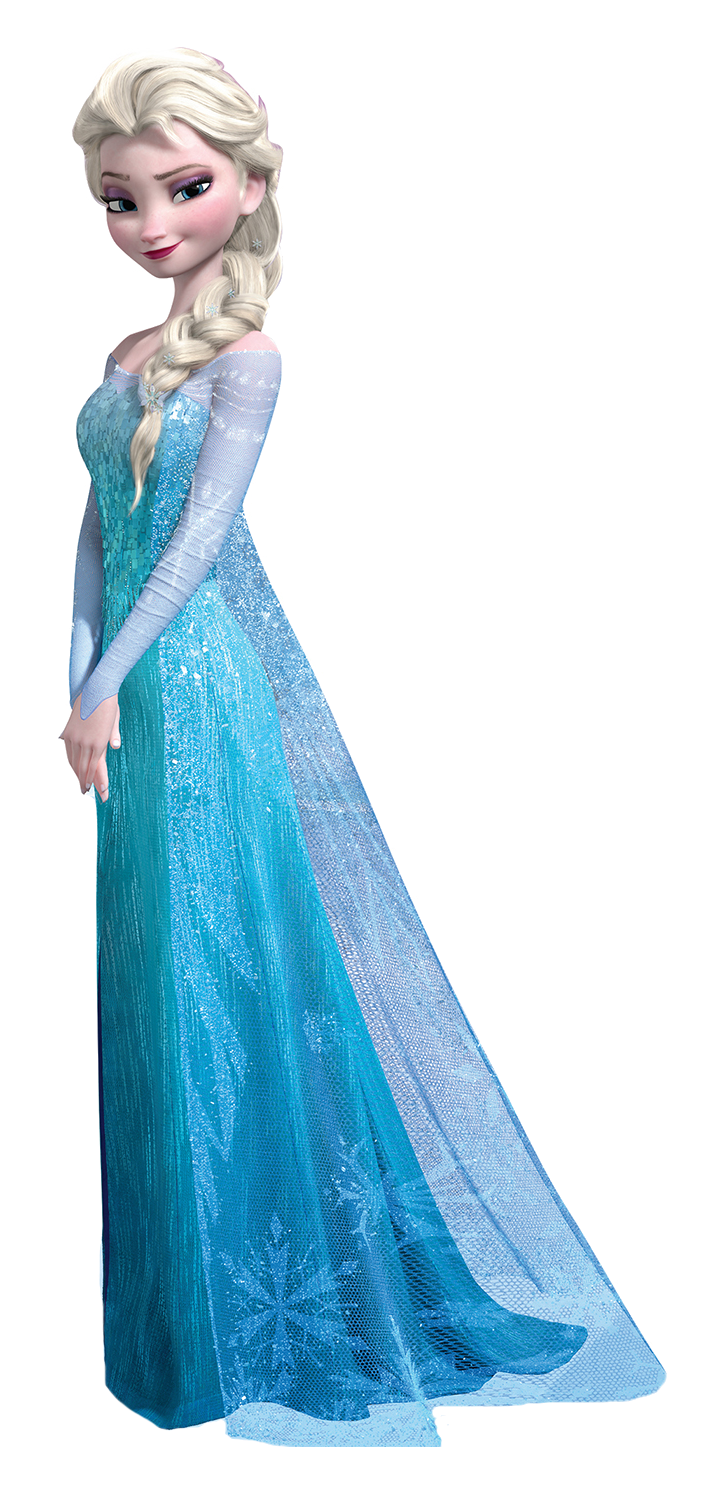
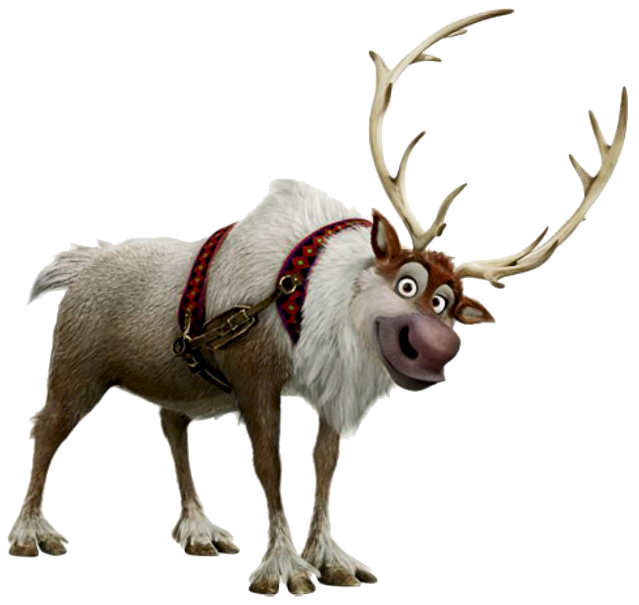
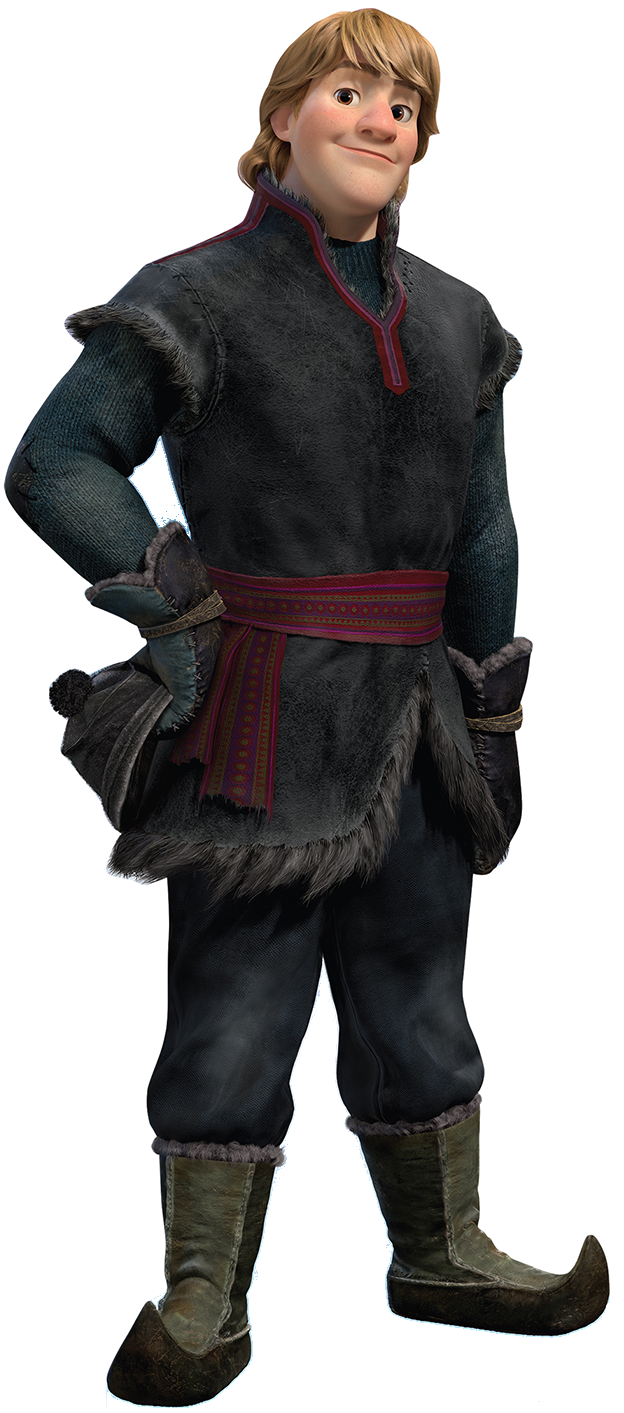
The DOM can also be accessed using Developer Tools or DevTools, these are built into most current browsers and really useful in inspecting, testing and debugging. This is just me messing around with the Google web page. Don't worry, changes made here are not permanent, the page goes back to it's original state when the page is refreshed.
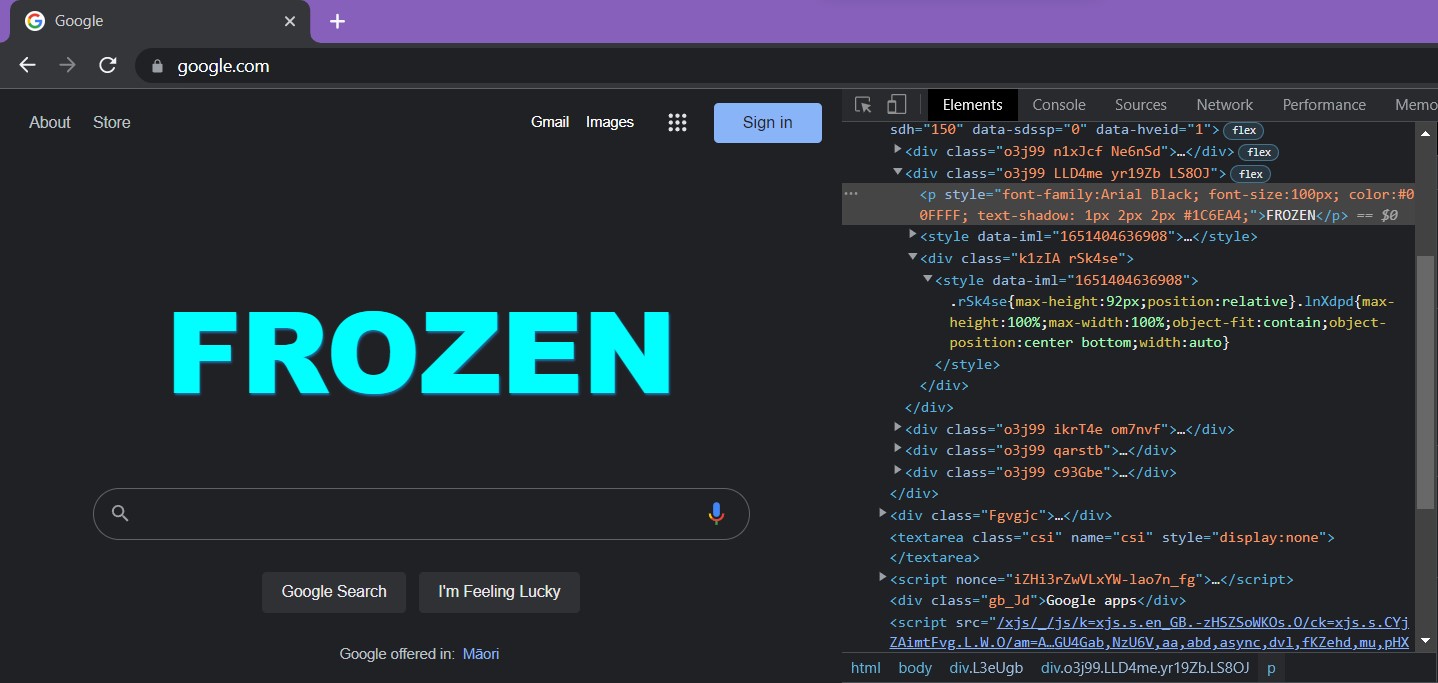
My husband says about 90% of the world's population (not fact checked nor verified) have seen Frozen so for the benefit of the 10%, my Frozen analogy stops here.
Control Flow, Loops & Functions
Control flow is the order that instructions in a program are executed. In JavaScript, this is from left-to-right, top-to-bottom unless it comes across another instruction that changes said flow. Consider the following code:
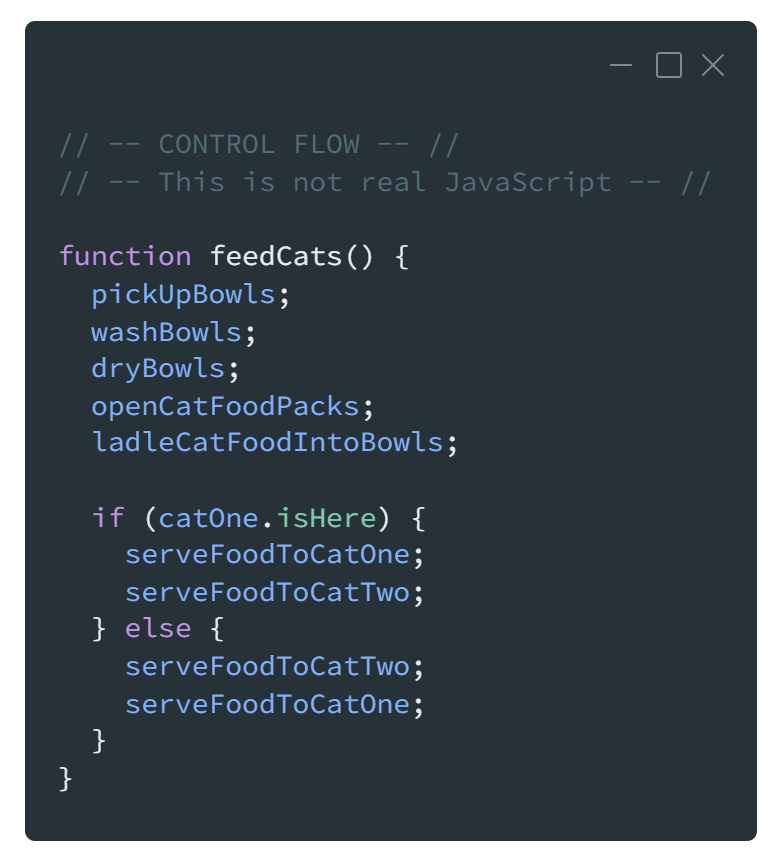
In order to feed my two cats correctly, I need to follow these steps in the correct order - can't really ladle food out of the pack if it ain't open. My cats have outdoor access and if catOne
(a.k.a. theDiva - not her real name) is indoors she always gets fed first; otherwise, I will never hear the end of it. If catTwo
is outside well, doesn't matter, catOne
has to be fed.
Regardless who gets fed first, it has to happen twice a day, so we setup a loop like so:
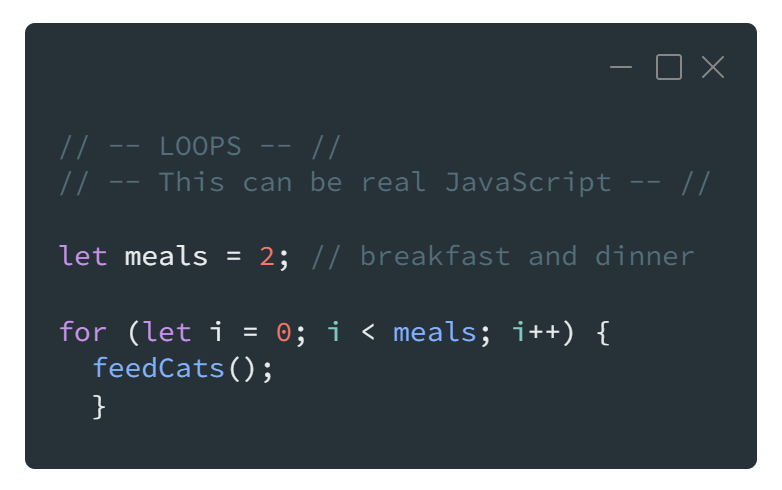
Notice how the function feedCats()
was just casually slipped into that loop? That's the beauty of functions - if I want to do something that's repetitive, I can just enclose the instructions (and conditionals or even more loops) into a function instead of typing them again and again when and where I want them to execute.
Arrays vs. Objects
An array is an ordered list of values that's stored in one variable. The values in the array don't have to be of the same type, they can be a mix of integers, strings or even objects. There are nine items in the following array but we start counting from zero hence each item is indexed as [0, 1, 2, 3, 4, 5, 6, 7, 8]
.

If I want to pick up the white kitty in the middle, we'd do it like this: let whiteKitty = cute[4];
We can now define whiteKitty
as an object.
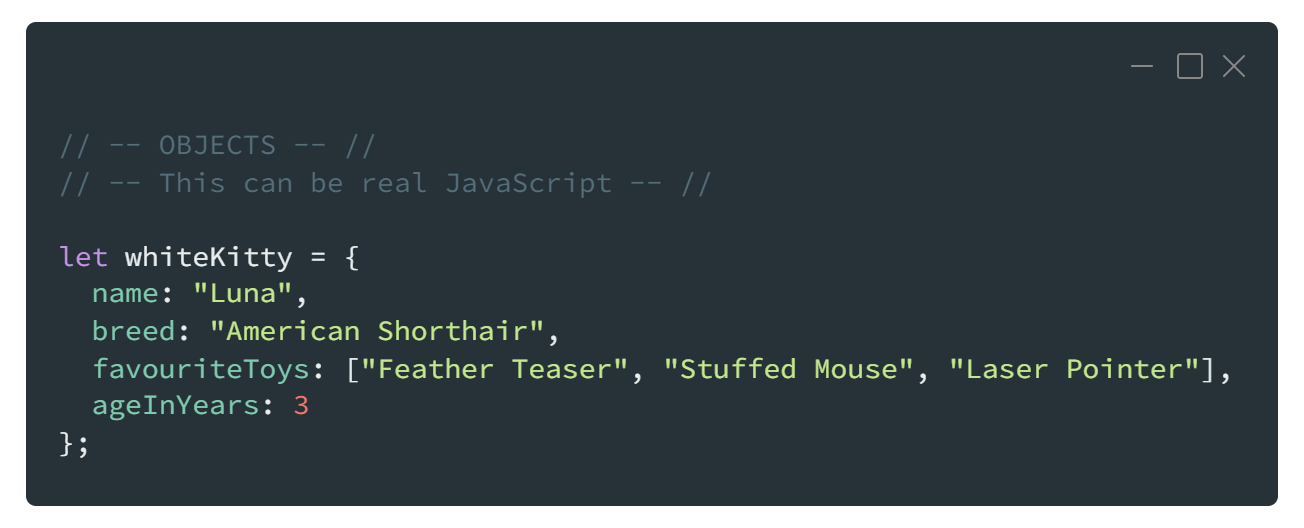
What are objects? Like an array, it's a collection, but of properties. Each of the items in our array cute
can be an object with it's own set of properties that may be different or not with another's. A property defines a characteristic of an object and consists of a key and a value (for example, name and Luna respectively). These properties can be accessed using .DOT or [ ] bracket notation.
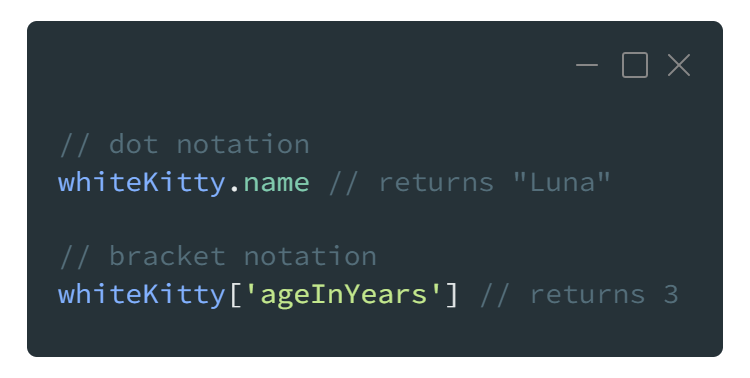
Let's say our beloved Luna no longer likes playing with her Laser Pointer. There are a lot of ways to remove this from the array of toys and here is one of them: whiteKitty.favouriteToys.splice(2, 2);
Phew, that is a lot of information to take in but it's just to give you an overview of how powerful these tools can be! Whenever you're ready to dive in, MDN Web Docs is a great resource.